High Java CPU usage due to string concatenation: String concat(+) vs. StringBuffer or StringBuilder append()
- package test;
- public class StrVsBuffVsBuild {
- public static void main(String[] args) {
- int count=200000;
- System.out.println("Number of Strings concat Operation is \'"+count+"\'");
- long st = System.currentTimeMillis();
- String str = "";
- for (int i = 0; i < count; i++) {
- str += "MyString";
- }
- System.out.println("Time taken for String concat (+) Operation is \'"+(System.currentTimeMillis()-st)+"\' Millis");
- st = System.currentTimeMillis();
- StringBuffer sb = new StringBuffer();
- for (int i = 0; i < count; i++) {
- sb.append("MyString");
- }
- System.out.println("Time taken for StringBuffer.append() Operation is \'"+(System.currentTimeMillis()-st)+"\' Millis");
- st = System.currentTimeMillis();
- StringBuilder sbr = new StringBuilder();
- for (int i = 0; i < count; i++) {
- sbr.append("MyString");
- }
- System.out.println("Time taken for StringBuilder.append() Operation is \'"+(System.currentTimeMillis()-st)+"\' Millis");
- }
- }
The String concat (+) method took 6.2 minutes, while StringBuffer and StringBuilder append() took only 19 and 5 milliseconds, respectively. Is it comparable? Moreover, during the entire 6.2-minute execution of the String concat() operation, the Java process took 100% CPU usage. You can see the CPU usage of the m/c and Java program in the graphs below:Number of Strings concat Operation is '200000' Time taken for String concat (+) Operation is '373933' Millis Time taken for StringBuffer.append() Operation is '19' MillisTime taken for StringBuilder.append() Operation is '5' Millis
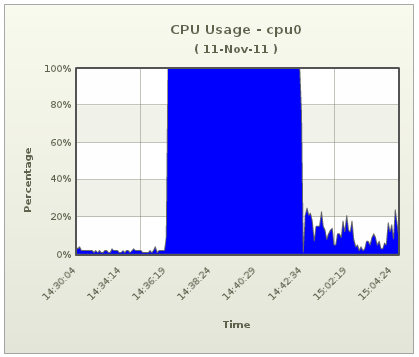
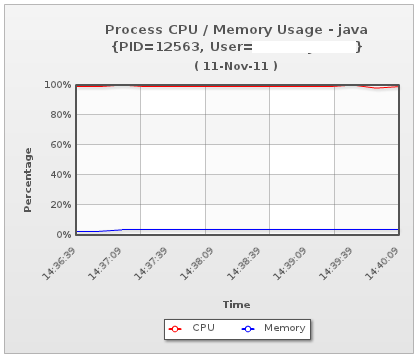
So, use StringBuilder or StringBuffer append() instead of String concat(+). Your next question might be "Which one is better, StringBuffer or StringBuilder?" Since the StringBuffer methods are synchronized, it is safe if the code is accessed by multiple threads. The StringBuilder methods are not synchronized, so it is not thread-safe. So if you need to append a global variable that can be accessed by multiple threads, you should use StringBuffer. If it is a method-level variable that cannot be accessed by multiple threads, you should use StringBuilder. Due to the absence of synchronization, StringBuilder is faster than StringBuffer.
Good Analysis.Actually StringBuilder is just a copy of StringBuffer without synchronization, if you look code on StringBuilder class you will realize that. by the way here are few more difference between StringBuilder and StringBuffer